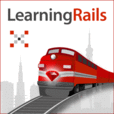
Summary: To listen to the lesson, click the Play button on the left. You can also right-click on the download link to save the mp3 file, or you can subscribe in iTunes (just search for Learning Rails). <p> </p> <p>To read a transcript of the lesson, click the Transcript link on the left.</p> <p><strong>The heart of the lesson is the audio</strong>; these notes are supplementary. So please listen to the audio, or read the transcript, before making use of these notes.</p> <h2>Lesson Notes</h2> <p><span class="caps">CRUD</span> is the acronym for the four main things you do with database records:</p> <ul> <li>Create</li> <li>Read</li> <li>Update</li> <li>Delete</li> </ul> <p>These notes illustrate the code described in the podcast. See the transcript for more details.</p> <p>For more details about forms processing with Rails, see the <a href="/topic/24227-creating-forms-with-ruby-on-rails">forms</a> page in our topics section. You’ll find some great screencasts listed there that will go into much more depth than this podcast.</p> <p>For a database of podcast episodes, with a title and a description field for each, the basic form code looks like this:</p> <pre> <% form_for @podcast do |form| %> <%= form.text_field :title %> <%= form.text_area :description %> <% end %> </pre> <p>The minimal code for the controller’s create action is:</p> <pre> Podcast.create params[:podcast] </pre> <p>The create method takes the parameters from the params[:podcast] hash, uses them to initialize a new object, and then saves that object to the database.</p> <p>This line to the podcast model ensures that podcasts can’t be saved with a blank title:</p> <pre> validates_presence_of :title </pre> <p>In the controller, the create action tests the value returned from the Podcast.create call, and if it is not false, it knows the save succeeded. If it is false, then the form is rendered again.</p> <pre> if Podcast.create params[:podcast] redirect_to (wherever you want to go after a successful save) else render :action => :new end </pre> <p>To display the error messages, the form view just includes a call to the error_messages_for helper:</p> <pre> error_messages_for :podcast </pre> <p>To make this an Ajax form, we can simply change the form_for helper to remote_form_for:</p> <pre> <% remote_form_for @podcast do |form| %> </pre> <p>The rest of the form remains the same. The controller and views must be modified to return just an <span class="caps">HTML</span> or JavaScript snipped, rather than an entire page, after a create or update action.</p>