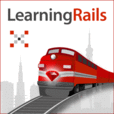
Summary: <h2>Goals</h2> <p>In this lesson, we put our application into a version control system, in preparation for deploying the application in the next lesson. We do this first because the proper way to deploy an application is not to copy it from your computer to the server, but to have the server pull the application from the version control system. Please note that, while we’ve tried to make these notes complete, they aren’t the full tutorial; that’s in the screencast, which you can access via the link on the left.</p> <h2>Choosing a version control system</h2> <p>There are many version control systems. <span class="caps">CVS</span> was the first system widely used in the open-source community. Several years ago, it was largely replaced by Subversion. And in early 2008, most of the Rails world moved to a newer version control system, called git. We’re going to use git in this lesson, since it has become the standard in the Rails community, and is used for Rails itself and most plugins.</p> <p>git was originally written by Linus Torvalds to manage development work on the Linux kernel. It’s an extremely capable system, with more than 100 commands. We’re going to just scratch the surface of its capabilities in this lesson, but you’ll learn enough to use it for day-to-day development. To make full use of it, spend some time with the resources listed on our <a href="/topic/24425-the-git-distributed-version-control-system">git page</a>. In particular, you may want to purchase the <a href="http://peepcode.com/products/git">PeepCode git screencast</a>.</p> <p>If you’re familiar with other version control systems, you probably expect there to be one central repository stored on a remote server somewhere. git is different; it is a distributed version control system, in which there can be any number of repositories for a single project. You’ll create a local repository on your computer, and if you want, you can create a remote repository elsewhere, such as on a hosted git service like github.com. (You’ll need to have a repository that is accessible from the Internet to deploy your application.)</p> <h2>Setup</h2> <p>We aren’t making any changes to the code in this lesson, and you don’t need to have the code installed locally to complete the lesson. If you follow along, by the end of the lesson you’ll have your own copy of the code on your computer, managed by a local git repository, and you’ll have your own repository stored on github as well.</p> <p>First we need to install git. Assuming you’re on a Mac and have Macports installed, open a terminal and enter:</p> <pre> sudo port install git-core </pre> <p>Now set up your user information in the git configuration:</p> <pre> git config --global user.name "Michael Slater" git config --global user.email learningrails@buildingwebapps.com </pre> <p>See our articles on installing Ruby on Rails for more details:</p> <ul> <li><a href="/articles/6433-setting-up-rails-on-leopard-mac" title="Mac OS X 10.5">Setting up Rails on Leopard</a></li> <li><a href="/articles/6455-setting-up-rails-on-tiger-mac" title="Mac OS X 10.4">Setting up Rails on Tiger</a></li> <li><a href="/articles/6491-setting-up-rails-on-windows-vista">Setting up Rails on Windows Vista</a></li> <li><a href="/articles/6467-setting-up-rails-on-windows-xp">Setting up Rails on Windows XP</a></li> </ul> <h2>Creating a git repository for an application on your computer</h2> <p>There’s two paths you could take to creating a repository on your computer:</p> <ul> <li>If you have an application already on your computer, you can create a local git repository and add the application to it</li> <li>If you want to start from an existing application, such as the Learning Rails sample application, you can make your own copy of that repository.</li> </ul> <p>In this lesson, we’ll do both of these things. First, we’ll show how we put the sample application into a git repository and then created a public repository on github. Then we’ll show how you can make your own version of that repository.</p> <p>Change to the root directory for the application and enter:</p> <pre> git init </pre> <p>This creates the .git folder at the root of your application, where git stores all of its information.</p> <h2>Telling git to ignore files we don’t want it to track</h2> <p>We don’t want git to track our log or temp files, so we need to create a file call .gitignore at the root of the application. If you’re using textmate, you can enter in the terminal:</p> <pre> mate .gitignore </pre> <p>And then enter the following lines into this file:</p> <pre> *.log tmp/**/* .DS_Store </pre> <p>The first line tells git to ignore any files that end with the .log extension. The second line tells it to ignore the temp directory contents (individual files as well as subdirectories), and the last line tells it to ignore the OS X housekeeping hidden file that is in each directory. (If you’re on Windows, you might want to replace this with Thumbs.db.)</p> <p>Now there’s one more little complexity with the ignore. Since we’ve told git to ignore all .log files, it sees the log directory as empty, and git doesn’t track empty directories. As a result, the log directory would not be put in the repository, and if you just checked out the files, you’d get an error on starting the application because Rails wouldn’t be able to create the log file. So we put a dummy file into the log directory, so git will not see the directory as empty and will put it in the repository.</p> <pre> touch log/.gitignore </pre> <h2>Adding files to our local git repository</h2> <p>Now we’re ready to add files to the repository. From the root of the application, enter:</p> <pre> git add . </pre> <p>which tells git to add all the files (except those excluded by our .gitignore file). Note that this doesn’t actually commit the files to the repository; it just adds them to what git calls the index, which lists files that are ready to be committed. You can view the list by typing:</p> <pre> git status </pre> <p>Now we can commit the files to the local repository by typing:</p> <pre> git commit -m "Initial commit" </pre> <p>The -m option allows us to specify a commit message, which is required. (If you leave off the -m option, git will try to open an editor for you to provide the commit message, but this will only work if you have the proper environment variable set up.)</p> <h2>Setting up a github account</h2> <p>We now have our application in our local repository. At this point we have all the benefits of version control, but we still want to copy this to a remote repository. There’s two reasons for this: first, as a backup, and second, so that other contributors, and most immediately, our deployment code (which we’ll get to in the next lesson) can access it.</p> <p>First, we need to create an account on <a href="http://github.com">github</a>. We’re going to create a temporary account for the purpose of this lesson. You’ll need to create your own if you want to have your own repository there.</p> <p>Visit www.github.com and click the Pricing and Signup link at the top. You can choose the free account as long as you’re willing for your code to be public; if you want a private repository, you’ll have to choose one of the paid accounts.</p> <h2>Setting up your <span class="caps">SSH</span> key</h2> <p>You’ll need an <span class="caps">SSH</span> key to use your repository. If you already have a key pair you’ve made for other purposes, you should be able to use your existing public key. If not, go ahead and create one now.</p> <p>For details on creating an <span class="caps">SSH</span> key, see the <a href="http://github.com/guides/providing-your-ssh-key">github guide on <span class="caps">SSH</span> keys</a>. If you’re on Windows, you may also find <a href="/articles/6397-using-ssh-keys-to-speed-login">our article on <span class="caps">SSH</span> with Windows</a> to be useful.</p> <p>Whether you’re using an existing key or created a new one, paste the public key into the signup form.</p> <h2>Push to the remote repository</h2> <p>The following command tells git that we want to add a remote repository definition, called origin by default, and provides the <span class="caps">URL</span> to that repository. (Note that we’ve shown the address for our learningrails repository at github here; you won’t be able to push to this repository, since you don’t have write access rights. We’ll show how you can push your version to your own remote repository shortly.)</p> <pre> git remote add origin git@github.com:learningrails/learning-rails-sample-app.git </pre> <p>And now we can copy to the remote repository with the command:</p> <pre> git push origin master </pre> <p>This tells git to push all changes in the local repository to the remote repository named <em>origin</em>, and to use the <em>master</em> branch (which in our case is the only branch that exists).</p> <p>When this completes, we have synchronized our local and remote repositories.</p> <h2>Making our own repository to hold your own version of the Learning Rails code</h2> <p>Now that you’ve seen how to create a repository from scratch, let’s make your own fork of the Learning Rails repository so you can use it to maintain your own version. In the next lesson, we’ll show how to deploy your application from that repository.</p> <p>First, you need to create an account at github, if you haven’t done that already, and log in.</p> <p>Once your account is set up, browse to http://github.com/mzslater/learning-rails-sample-app/tree/master (or search for learning-rails-sample-app from the github search facility, but make sure you choose the version whose owner is mzslater).</p> <p>Click the Fork button, and github will create a repository that is a duplicate of our repository, and it also keeps track of the relationship between the two repositories.</p> <h2>Cloning your repository</h2> <p>You now have your own repository! The next step is to clone it on your local machine.</p> <p>On your computer, open a terminal and change to the directory in which you want to place your code. We use a directory called “development” in our user directory. You don’t need to make a subdirectory for the application; git will do that for you.</p> <p>On your repository page on github, you’ll see the clone <span class="caps">URL</span> listed, and if you click it github will display the full command that you need to enter on your computer to create the clone. It will be something like:</p> <pre> git clone git://github.com/accountname/learning-rails-sample-app.git </pre> <p>where accountname is the name you chose when you created your github account.</p> <p>Run this command, and if all goes well, you’ll have a local clone of your github repository.</p> <p>If you get a permission denied message, you probably have a problem with your ssh key. Check <a href="http://github.com/guides/addressing-authentication-problems-with-ssh">this page</a> for some pointers.</p> <h2>Changing your version of the code</h2> <p>Now make some edit to your local code, so you can test the process of committing changes and pushing them up to the github repository.</p> <p>After making the change, enter the command:</p> <pre> git commit -a -m 'make up a commit message here' </pre> <p>Your change is now committed to your local repository, but the github repository hasn’t yet been updated. To push the change up to that repository, enter the command:</p> <pre> git push origin master </pre> <p>Now you can visit your repository at github, and it will show the commit. You can click on the commit to see the changes that were made, and use the source code browser to see all the code.</p> <p>If you make improvements to the code that you think would be of interest to others, click the Pull Request button on your repository page to send us a message telling us what you’ve done, and we’ll consider adding it to our repository. Please be specific about describing the changes you’ve made and why they are useful to everyone.</p> <h2>Coming Up</h2> <p>In our next lesson, we’ll explore the Capistrano deployment utility and deploy the code from the github repository.</p>